배열이란?
배열(array)이란 연관된 데이터를 모아서 통으로 관리하기 위해서 사용하는 데이터 타입이다.
변수가 하나의 데이터를 저장하기 위한 것이라면 배열은 여러 개의 데이터를 하나의 변수에 저장하기 위한 것이라고 할 수 있다.
● 동일한 자료형의 순차적 자료 구조
● 인덱스 연산자[]를 이용하여 빠른 참조가 가능
● 물리적 위치와 논리적 위치가 동일
● 배열의 순서는 0부터 시작
● 자바에서는 객체 배열을 구현한 ArrayList를 많이 활용함
배열 선언과 초기화
배열 선언하기
int[] arr1 = new int[10];
int arr2[] = new int[10];
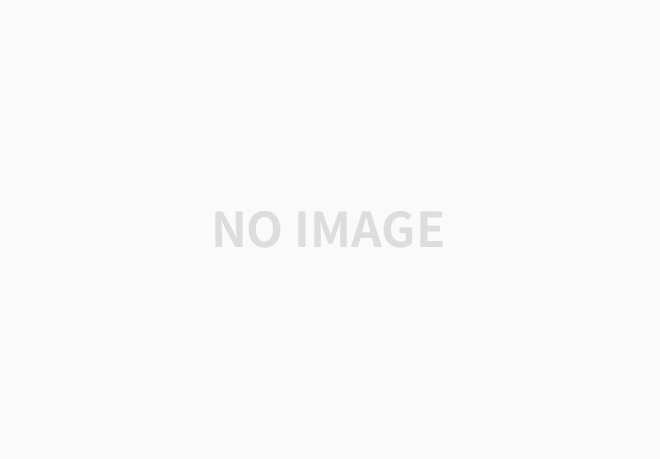
배열요소의 개수 .length
개수 정하고 바로 초깃값 주면 오류
int[ ] g = new int[5] {10, 20, 30, 40, 50} 오류
배열 예제
public class ArrayMainTest1 {
public static void main(String[] args) {
// 배열이란
// ** 연관된 데이터를 모아서 통으로 관리하기 위해 사용하는 데이터 타입이다. **
int[] arr1; // 변수의 선언 (int가 아니라 int 배열입니다.)
arr1 = new int[2]; // 배열의 초기화는 반드시 크기를 먼저 지정해주어야 사용 가능하다 8바이트
// 배열의 크기도 생각을 해보자
double[] arr2 = new double[2]; // 선언과 동시에 초기화 16byte
// 값을 넣는 방법
arr1[0] = 100; // [인덱스] 0부터 시작
arr1[1] = 1000;
// 인덱스의 순서는 0번부터 시작한다.
System.out.println(arr1[1]);
System.out.println(arr1[0]);
// 주의 : 배열의 길이와(크기)와 인덱스의 번호는 다르다
// 공식 --> 배열의 인덱스의 번호는 n-1
// System.out.println(arr1[2]); <--- 오류 발생
arr2[0] = 10.0;
arr2[1] = 20;
arr2[0] = 0.0;
System.out.println(arr2[0]);
System.out.println(arr2[1]);
} // end of main
}
결과
1000
100
0.0
20.0
배열 예제2 book
클래스
package ch09;
public class Book {
private String title;
private String author;
private int totalPage;
public Book(String title, String author) {
super();
this.title = title;
this.author = author;
}
public Book(String title, String author, int totalPage) {
this(title, author);
this.totalPage = totalPage;
}
public void showInfo() {
System.out.println(">>>책정보<<<");
System.out.println("제목 : " + this.title);
System.out.println("작가 : " + this.author);
System.out.println("--------------------");
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getTotalPage() {
return totalPage;
}
public void setTotalPage(int totalPage) {
this.totalPage = totalPage;
}
}
객체
package ch09;
public class BookMainTest1 {
public static void main(String[] args) {
// Book book1 = new Book("홍길동전", "허균");
// 연관된 데이터를 통으로 모아서 관리하고 싶다면 배열 - 자료구조 사용하자
Book[] arrBooks = new Book[10]; // 배열은 반드시 먼저 크기를 지정해야 한다.
// arrBooks[0] = 10; <-- 오류 발생
arrBooks[0] = new Book("플러터UI실전", "김근호");
arrBooks[1] = new Book("무궁화꽃이피었습니다", "김진명");
arrBooks[2] = new Book("흐르는강물처럼", "파울로코엘료");
arrBooks[3] = new Book("리딩으로리드하라", "이지성");
arrBooks[4] = new Book("사피엔스", "유발하라리");
// 배열의 크기는 10 이다. 인덱스 마지막 번호 9 이다.
// 배열은 보통 반복문과 함께 많이 사용 된다.
// 배열의 인덱스로 접근해서 .연사자를 통해 객체의 기능을 호출할 수 있다.
arrBooks[2].showInfo();
// 10 --> 코드로 치환 하자.
System.out.println("배열의 길이를 알려주는 변수 : " + arrBooks.length);
for (int i = 0; i < arrBooks.length; i++) {
// 반복문에서는 배열을 어떻게 활용해야 할까?
// i -- 0
// i -- 1
// i -- 2 ...
// 방어적 코드를 작성해 보자
if (arrBooks[i] != null) {
arrBooks[i].showInfo();
System.out.println();
}
// arrBooks[i].showInfo();
}
System.out.println("-----------------");
// 주의 하자 NullPointerException !!
// 딱 2가지 기억
// 1. 객체를 생성하지 않았거나
// 2. 변수에 주소값을 할당하지 않았거나
// 배열의 길이와(크기) 안에 들어가 있는 값의 개수는 다를 수 있다.
// 정리
// 1. 인덱스의 크기는 n - 1 개이다.
// 2. 배열의 길이와 실제로 들어가 있는 값의 개수는 다를 수 있다
// 3. 배열에는 연관된 데이터만 통으로 모아서 관리할 수 있다
// 4. 배열은 반복문과 함께 많이 사용한다. - 오류 발생 - 방어적 코드 작성
} // end of main
} // end of class
결과
>>>책정보<<<
제목 : 흐르는강물처럼
작가 : 파울로코엘료
--------------------
배열의 길이를 알려주는 변수 : 10
>>>책정보<<<
제목 : 플러터UI실전
작가 : 김근호
--------------------
>>>책정보<<<
제목 : 무궁화꽃이피었습니다
작가 : 김진명
--------------------
>>>책정보<<<
제목 : 흐르는강물처럼
작가 : 파울로코엘료
--------------------
>>>책정보<<<
제목 : 리딩으로리드하라
작가 : 이지성
--------------------
>>>책정보<<<
제목 : 사피엔스
작가 : 유발하라리
--------------------
-----------------
tencobooks
package com.tenco.books;
import java.util.Scanner;
import ch09.Book;
/**
* @author 도권재
* 책을 관리하는 프로그램
* C R U D 기능을 구현해보자
*/
public class TencoBooks {
public static void main(String[] args) {
// 준비물
Scanner sc = new Scanner(System.in);
Book[] books = new Book[100];
final String SAVE = "1";
final String SEARCH_ALL = "2";
final String SEARCH_TITLE = "3";
final String DELETE_ALL = "4";
final String UPDATE = "5";
final String END = "0";
boolean flag = true;
// 마지막에 저장된 인덱스 번호를 기억해 두자.
int lastIndexNumber = 0;
// todo 샘플 데이터 추후 삭제
books[0] = new Book("홍길동전","허균");
books[1] = new Book("사피엔스","유발하라리");
lastIndexNumber = 2;
while(flag) {
System.out.println("** 메뉴 선택 **");
System.out.println("1. 저장 2. 전체조회 3. 선택조회 4. 전체삭제 5.책 정보 수정 0. 종료");
System.out.println("-----------------------------------");
String selectedNumber = sc.nextLine();
// 숫자는 == 문자는 .equals
if(selectedNumber.equals(END)) {
flag = false;
} else if(selectedNumber.equals(SAVE)) {
lastIndexNumber = save(sc, books, lastIndexNumber);
} else if(selectedNumber.equals(SEARCH_ALL)) {
searchAll(books);
} else if(selectedNumber.equals(SEARCH_TITLE)) {
searchTitle(sc,books);
} else if(selectedNumber.equals(DELETE_ALL)) {
deleteAll(sc,books);
} else if(selectedNumber.equals(UPDATE)) {
update(sc,books);
} else {
System.out.println("잘못 입력 하셨습니다. 다시 선택해주세요");
}
} // end of while
System.out.println("프로그램이 종료 되었습니다");
} // end of main
// 저장하는 기능 static 메서드로 만들어 보기
public static int save(Scanner scanner, Book[] books, int index) {
System.out.println(">>저장하기<<");
System.out.println("책 제목을 입력하세요");
String bookTitle = scanner.nextLine();
System.out.println("저자 이름을 입력하세요");
String bookAuthor = scanner.nextLine();
Book book = new Book(bookTitle, bookAuthor);
books[index] = book;
index++;
System.out.println(bookTitle + " 책을 생성 했습니다.");
return index;
}
// 전체 조회
public static void searchAll(Book[] books) {
System.out.println(">> 전체 조회 하기 <<");
for(int i = 0; i < books.length; i++) {
if(books[i] != null) {
books[i].showInfo();
System.out.println("-------------");
}
}
}
// 선택 조회 - 책 제목으로 검색 하기
public static void searchTitle(Scanner scanner, Book[] books) {
System.out.println(">> 선택 조회하기 <<");
String bookTitle = scanner.nextLine();
for(int i = 0; i < books.length; i++) {
if(books[i] != null) { // 방어적 코드 작성
// "홍길동전.equals("사용자가 입력한 값)"
if(books[i].getTitle().equals(bookTitle)) {
//같은 책 제목이 존재 한다 ! <-- true
books[i].showInfo();
break; // 여기서는 선택 조회라 데이터를 찾았다면 굳이 모든 반목문을 동작 시킬 필요가 없다.
}
}
}
}
public static void deleteAll(Scanner scanner, Book[] books) {
System.out.println(">> 전체 삭제하기 <<");
String booktitle = scanner.nextLine();
for(int i=0; i<books.length; i++) {
if(books[i].getTitle().equals(booktitle)) {
//books[i] = null;
books[i].setTitle(null);
books[i].setAuthor(null);
}
}
}
public static void update(Scanner scanner, Book[] books) {
System.out.println(">>책 정보 수정하기<<");
String booktitle = scanner.nextLine();
for(int i=0; i<books.length; i++) {
if(books[i]!=null) {
if(books[i].getTitle().equals(booktitle)) {
System.out.println("바꿀 책 제목");
String a = scanner.nextLine();
System.out.println("바꿀 저자 이름");
String b = scanner.nextLine();
books[i].setTitle(a);
books[i].setAuthor(b);
books[i].showInfo();
}
}
}
}
} /
결과
'JAVA' 카테고리의 다른 글
[JAVA] 다형성 (0) | 2023.08.09 |
---|---|
[JAVA] 상속 (0) | 2023.08.07 |
[JAVA] this 사용법과 스타크래프트 게임 만들기 그리고 CRUD (0) | 2023.08.02 |
[JAVA] 접근 제어 지시자 (0) | 2023.08.01 |
[JAVA] 생성자를 사용하여 클래스 설계하여 객체 생성 (0) | 2023.07.31 |